My favorite Laravel's Blade Tricks
Published on August 02, 2020
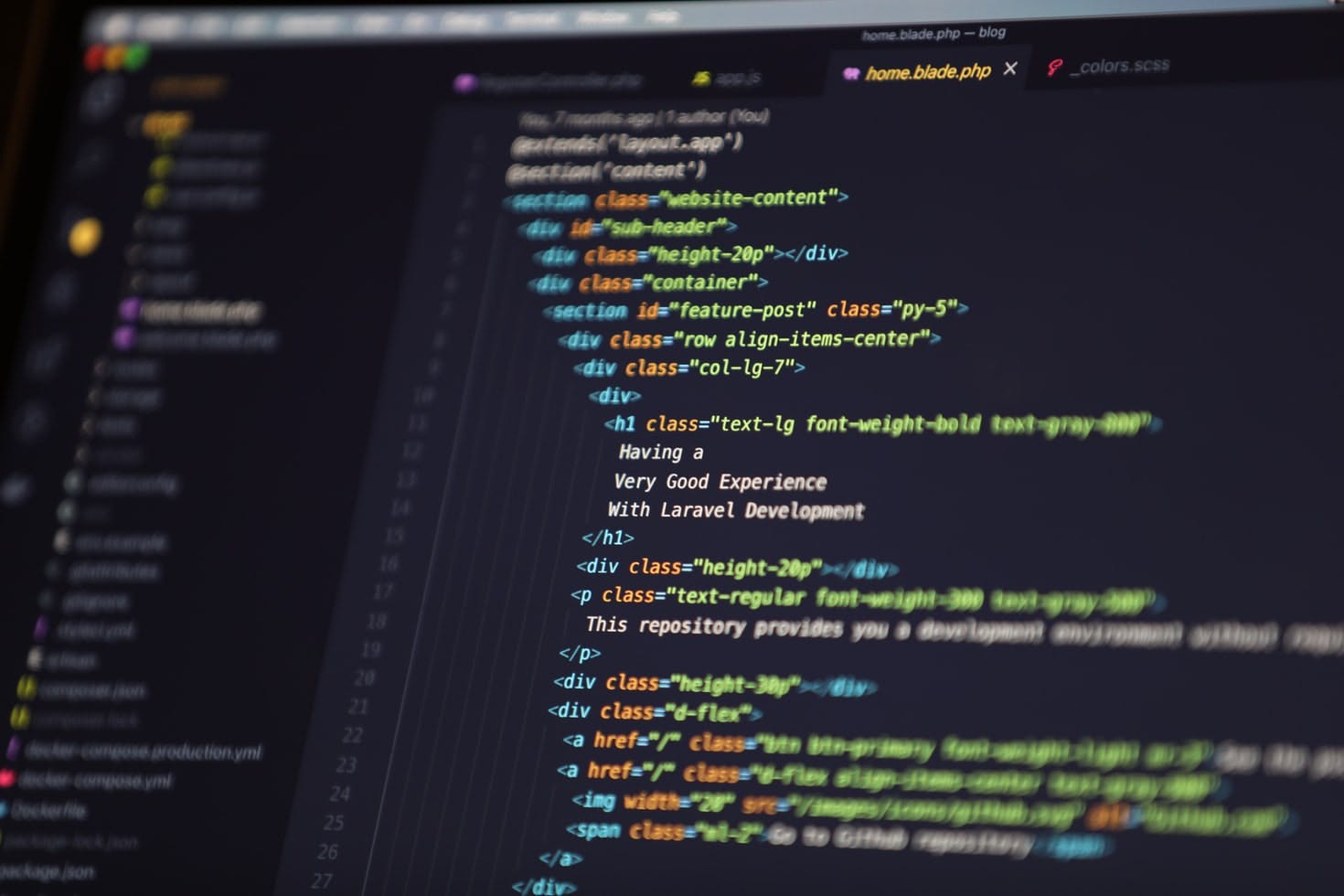
Beside the ability to make inheritance template using @extends, @section, and @yield, Laravel also let us to write html with any condition that we desire according to our application. Here are some of my favorite Laravel’s blade trick to make the development process faster and easier.
@if
This directive is useful when we’re trying to display data in view on various condition. We can use as many as @elseif that we need in Laravel’s view, but keep in mind of your website performance.
1@if (count($data) === 1)
2<p>You only have one data</p>
3@elseif (count($data) > 1)
4<p>You have more than one data</p>
5@else
6<p>You don't have any data</p>
7@endif
@auth and @guest
We can use @auth and @guest usually to check either the user is authenticated or not to display the appropriate button, for example to display button Login for unauthenticated user and button Logout for the authenticated user.
1@auth
2<button>Logout</button>
3@endauth
4
5@guest
6<button>Login</button>
7@endguest
@for
If we want to do a simple loop in Laravel’s blade, we can use @for just like PHP’s loop structures.
1@for ($i = 0; $i <= 10; $i++)
2The current order is {{$i}}
3@endfor
@foreach
When we display a list of data in the view, we can use @ foreach to loop through the variable to get each value.
1@foreach ($users as $user)
2<tr>
3<td>{{$user->name}}</td>
4<td>{{$user->email}}</td>
5</tr>
6@endforeach
@forelse
This directive is use to handle if the variable that we pass is empty to let the user know that there is no data to show.
1@forelse ($users as $user)
2<tr>
3<td>{{$user->name}}</td>
4<td>{{$user->email}}</td>
5</tr>
6@empty
7<tr colspan="2">
8<td>There is no user</td>
9</tr>
10@endforelse
@loop
When we do a loop, variable $loop will be available inside the loop. This variable provide many useful information, my favorite one is $loop->iteration to generate serial row number in table.
1@foreach ($users as $user)
2<tr>
3<td>{{$loop->iteration}}</td>
4<td>{{$user->name}}</td>
5</tr>
6@endforeach
For the list of available properties, you can check Laravel’s documentation
@csrf
Anytime we use a form in Laravel, we need to include CSRF token field in the form for security purpose. We may use @csrf directive to generate it.
1<form method="POST" action="/user">
2@csrf
3...
4</form>
@method
In Laravel we can use PUT, PATCH, or DELETE request. But html form not support that method, so we need to add hidden _method field. We can use @method to create that.
1<form action="/user" method="POST">
2@method('PUT')
3...
4</form>
@error
We need to provide error handling in every request that user make, especially when submitting a form. We can use @ error to make a place in our view to display error message to the user.
1@error('title')
2<div class="alert alert-danger">{{ $message }}</div>
3@enderror
That are some of the trick that I like to use when developing using Laravel. What’s your favorite tricks? Let me know in the comment section, and I planned to make more article about Laravel like this. Thank you for reading!