Let's compare JSX with HTML
Published on September 30, 2021
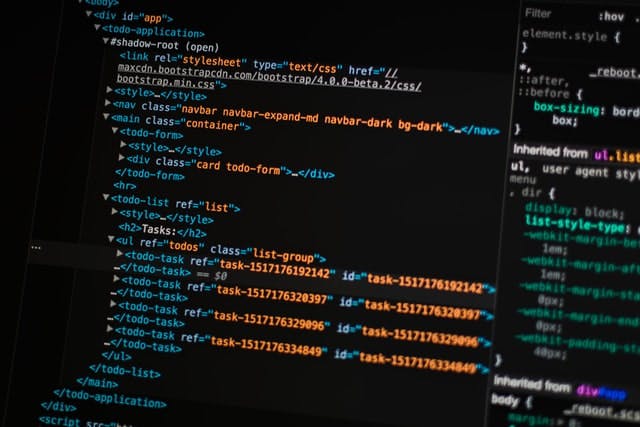
A web page is made of HTML as its structure, stands for Hypertext Markup Language. The code is either HTML originally or compile to it so the browser can read it and show the content of the web page on your screen.
On the other hands, JSX stands for JavaScript Syntax Extension or some called JavaScript XML. It was introduced with Reactjs around 2013. It allows developers to write HTML within JavaScript. So technically when you’re writing JSX, you’re writing JavaScript and HTML together. Also that’s why some HTML attribute renamed on JSX, like the “**class**
” attribute on HTML to be “**className**
” on JSX since “**class**
” is a common keyword for JavaScript.
Other thing is, HTML is natively supported by all browser. While JSX on the other hands, is not. So you need a compiler like Babel or Webpack to make the browser can understand it. Now let’s compare JSX with HTML
So, what the main difference?
JSX must return a single parent element
One major difference is you must return a single parent element in JSX, or it won’t compile. While on HTML you are free to do what ever you want as you don’t have to return a single parent element in a single file. Let’s see here, JSX give an error because there are two parent elements.
Error on JSX if there are more than one parent
To fix this, we can add fragment which is **<>...</>**
. See below after fragment added.
There’s no error after adding fragment
You can write JavaScript code directly inside JSX
In JSX, you can directly write JavaScript code alongside the html tag. Where in HTML you need a <script>
tag or an external JS file to implement JavaScript. In JSX you can write JavaScript code between curly braces {...}
. Like below:
1const App = () => {
2 return (
3 <>
4 {new Date()}
5 <p>Hi there!</p>
6 <p>How are you?</p>
7 </>
8 );
9};
export default App;
JSX knows className and HTMLFor, not class and for
In HTML you write class names and for attribute like so, **class**
and **for**
. But in JSX you don’t write those since both are reserved keyword in JavaScript, instead write **className**
and **HTMLFor**
.
1const App = () => {
2 return (
3 <div className="container">
4 {new Date()}
5 <p>Hi there!</p>
6 <p>How are you?</p>
7 <br />
8 <form>
9 <label htmlFor="name">Name</label>
10 <input type="text" />
11 </form>
12 </div>
13 );
14};
export default App;
JSX likes camelCase
In JSX, all HTML attributes and event references are written in camelCase. So, **onclick**
become **onClick**
, **onmouseover**
become **onMouseOver**
and so on.
1const App = () => {
2 const sayHi = () => {
3 alert("Hi there!");
4 };
5 return (
6 <div className="container">
7 {new Date()}
8 <p>Hi there!</p>
9 <p>How are you?</p>
10 <br />
11 <button onClick={sayHi}>Say it!</button>
12 </div>
13 );
14};
15
16export default App;
Inline styles are written as objects in JSX
While in HTML you write inline styling inside the tag as a string, in JSX the style is an object. So you define the style name with the properties in camelCase, the values in quotes, then you can pass it inline to the JSX.
1const App = () => {
2 const inlineStyle = {
3 color: "#000000",
4 fontSize: "12px"
5 };
6
7 return (
8 <div className="container">
9 {new Date()}
10 <p style={inlineStyle}>Hi there!</p>
11 <p>How are you?</p>
12 <br />
13 <button onClick={sayHi}>Say it!</button>
14 </div>
15 );
16};
17
18export default App;
or you could write the styles directly inside the JSX tag like this:
1const App = () => {
2 return (
3 <div className="container">
4 {new Date()}
5 <p style={{color: "#000000",fontSize: "12px"}}>Hi there!</p>
6 <p>How are you?</p>
7 <br />
8 <button onClick={sayHi}>Say it!</button>
9 </div>
10 );
11};
12
13export default App;
But of course it make your code cleaner by define the object outside JSX first and then pass it into the opening tag.
That’s it! Those are the differences that I realize when I’m working with JSX. Such a handy way to build a website! Thank you for reading, please leave a comment if you find this interesting and helpful.